The ASP.NET DataList control is a more customizable version of the GridView and a lightweight server-side control that serves as a container for data items. The DataList is used to display data in a list format but requires more manual work as you have to design it yourself. It does not support paging or sorting features, so you have to implement them manually.
The DataList control has four templates:
1. HeaderTemplate – The content of the HeaderTemplate template will not be rendered; this is the head section of the DataList.
2. ItemTemplate – The content of the ItemTemplate template will be rendered for each data item in the DataSource.
3. SeparatorTemplate – The SeparatorTemplate template is used to add a separator between two items in the DataList.
4. FooterTemplate – The content of the FooterTemplate template will not be repeated.
To begin, open Visual Studio and add a new empty website by navigating to File > New > Web Site, then select 'Empty Web Site'.
Now, I have added a DataList control on the page. I want to display the name and email of the user in the DataList control. I have added a table in the ItemTemplate which will be repeated for each item in the dataset.
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.auto-style1 {
width: 100px;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<asp:DataList ID="DataList1" runat="server" Width="518px">
<ItemTemplate>
<table class="auto-style1" style="width:100%">
<tr style="background-color: #666699; height: 50px;">
<td> Name <asp:Label ID="lblname" runat="server" style="float:right" Text='<%#Eval("name") %>'></asp:Label></td>
</tr>
<tr style="background-color: #FFCCFF;height: 50px;">
<td> Email<asp:Label ID="lblemail" runat="server" style="float:right" Text='<%#Eval("email") %>'></asp:Label></td>
</tr>
</table>
</ItemTemplate>
</asp:DataList>
</form>
</body>
</html>
Now, I have created a dummy table (for testing purposes) and added this table into a DataSet (a collection of tables). I have made this DataSet the data source for the DataList control.
Alternatively, you can also bind the DataList control directly from the database. However, for testing purposes, I have created a dummy DataSet.
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
BindDatalist();
}
public void BindDatalist()
{
DataSet ds = new DataSet();
DataTable dttable = new DataTable();
dttable.Columns.Add("name");
dttable.Columns.Add("email");
dttable.Rows.Add(new object[] {"jacob","[email protected]" });
dttable.Rows.Add(new object[] { "zoltan", "[email protected]" });
ds.Tables.Add(dttable);
DataList1.DataSourceID = null;
DataList1.DataSource = ds.Tables[0].DefaultView;
DataList1.DataBind();
}
}
run your application:-
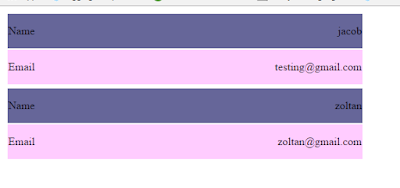
<asp:DataList ID="DataList1" runat="server" Width="518px">
<HeaderTemplate><asp:Label ID="lblname" runat="server" style="background-color:brown;height:80px;" Text="UserInformation"></asp:Label></HeaderTemplate>
<ItemTemplate>
<table class="auto-style1" style="width:100%">
<tr style="background-color: #666699; height: 50px;">
<td> Name <asp:Label ID="lblname" runat="server" style="float:right" Text='<%#Eval("name") %>'></asp:Label></td>
</tr>
<tr style="background-color: #FFCCFF;height: 50px;">
<td> Email<asp:Label ID="lblemail" runat="server" style="float:right" Text='<%#Eval("email") %>'></asp:Label></td>
</tr>
</table>
</ItemTemplate>
</asp:DataList>
DataSet and DataTable Initialization:
Adding Data to DataTable:
DataSet Configuration:
Data Binding:
A DataSet containing a DataTable with two columns ("name" and "email") and adds two rows of data to the DataTable. Then, it binds this data to a DataList control, displaying each row of data in a stylized format defined by the DataList's layout template.
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.auto-style1 {
width: 100px;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<asp:DataList ID="DataList1" runat="server" Width="518px" RepeatColumns="3">
<HeaderTemplate >UserInformation</HeaderTemplate>
<ItemTemplate>
<table class="auto-style1" style="width:100%">
<tr style="background-color: #666699; height: 50px;">
<td> Name <asp:Label ID="lblname" runat="server" style="float:right" Text='<%#Eval("name") %>'></asp:Label></td>
</tr>
<tr style="background-color: #FFCCFF;height: 50px;">
<td> Email<asp:Label ID="lblemail" runat="server" style="float:right" Text='<%#Eval("email") %>'></asp:Label></td>
</tr>
</table>
</ItemTemplate>
</asp:DataList>
</form>
</body>
</html>
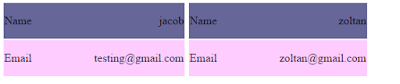
If you want to bind the DataList Control from database first create table tbluser in database.
CREATE TABLE [dbo].[tbluser](
[Userid] [int] IDENTITY(1,1) NOT NULL,
[Username] [varchar](100) NULL,
[password] [varchar](100) NULL,
[Address] [varchar](100) NULL
)
select * from tbluser
You want Bind user details to a DataList control then create connection string in the web.config
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.auto-style1 {
width: 100px;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<asp:DataList ID="DataList1" runat="server" Width="518px">
<ItemTemplate>
<table class="auto-style1" style="width:100%">
<tr style="background-color: #666699; height: 50px;">
<td> UserName <asp:Label ID="lblname" runat="server" style="float:right" Text='<%#Eval("Username") %>'></asp:Label></td>
</tr>
<tr style="background-color: #FFCCFF;height: 50px;">
<td> Userpassword<asp:Label ID="lblemail" runat="server" style="float:right" Text='<%#Eval("password") %>'></asp:Label></td>
</tr>
<tr style="background-color: #FFCCFF;height: 50px;">
<td> UserAddress<asp:Label ID="Label1" runat="server" style="float:right" Text='<%#Eval("Address") %>'></asp:Label></td>
</tr>
</table>
</ItemTemplate>
</asp:DataList>
</form>
</body>
</html>
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
BindDatalist();
}
public void BindDatalist()
{
SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["constr"].ToString());
SqlCommand cmd = new SqlCommand("Select * from tbluser", con);
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataSet ds = new DataSet();
da.Fill(ds);
if (ds.Tables[0].Rows.Count > 0)
{
DataList1.DataSourceID = null;
DataList1.DataSource = ds.Tables[0].DefaultView;
DataList1.DataBind();
}
}
}