In this post, we will learn how to send a file through the HTTP client in ASP.NET using C#. The concept is to be able to send any type of file, such as XLS, PDF, Word documents, etc., through the HTTP client class to a web API.
Before we proceed to code the solution for sending the file through the HTTP client, let me first show you the setup in my ASP.NET Core REST web API to receive the file.
In this post, we will cover the following points
- How to post file and data to api using httpclient c#
- HttpClient multipart/form-data upload
- How to send a file and form data with HttpClient in C#
- Upload Files with HttpClient to ASP.NET WebApi or Asp Core
- Multipart form-data in HttpClient Post REST API
So here we have a file rest Web API endpoint, which is an API that I designed specifically for this example. Here I have a post endpoint and as you can see, it accepts UserProfileModel model class as a parameter, and UserProfileModel has properties UserId, Name, Address, Description, and Image which is a representation of a file. And the name of this parameter is file.
And also we are using FromForm because in order to receive files I need to use FromForm I cannot use Frombody.
Now here what I’m doing is that I am using this service, which allows me to save the file into the webserver specifically in the www root directory.
REST API upload file example C#
[HttpPost] [Consumes("multipart/form-data")] public async Task<IActionResult> Profileupdate([FromForm] UserProfileModel userProfileModel) { ApiResponse resp = new ApiResponse(); resp.code = 100; if (!ModelState.IsValid) { resp.msg = Message.invalid_model; return Ok(resp); } try { var file = userProfileModel.Image; string file_Extension = Path.GetExtension(file.FileName); string filename = Guid.NewGuid() + "" + (!string.IsNullOrEmpty(file_Extension) ? file_Extension : ".jpg"); var uploadspath = Path.Combine(_hostingEnvironment.WebRootPath, "profilepicture") + "/" + userProfileModel.UserId + "/"; // Get the complete folder path and store the file inside it. using (var fileStream = new FileStream(Path.Combine(uploadspath, filename), FileMode.Create)) { await file.CopyToAsync(fileStream); } resp.code = 0; } catch (Exception ex) { } return Ok(resp); } public class UserProfileModel { public int UserId { get; set; } public string Name { get; set; } public string Address { get; set; } public string Description { get; set; } public IFormFile Image { get; set; } }
HTML for updating the user profile
Now let’s go to our Html page, which is our web application, and from here that we want to send a file with user data information.
<form> <fieldset> <legend>Post file and data to api using httpclient</legend> <div class="form-group"> <label for="txtName">Name</label> <input type="text" name="txtName" class="form-control" id="txtName" placeholder="Name" /> </div> <div class="form-group"> <label for="txtAddress">Address</label> <input type="text" name="Address" class="form-control" id="txtAddress" name="txtAddress" placeholder="Address" /> </div> <div class="form-group"> <label for="txtDescription">Description</label> <input type="text" name="txtDescription" class="form-control" id="txtDescription" placeholder="Description" /> </div> <div class="form-group"> <label for="fileupload">Profile Picture</label> <input type="file" class="form-control" id="fileupload" /> </div> <div class="form-group"> <input type="button" id="btnregistered" value="Update" /> </div> </fieldset> </form>
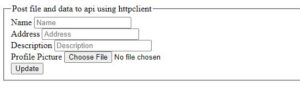
Send a image file and form data with HttpClient
and Onclick submit button we are calling this action method. using below code you can Post file as well as some parameter to web api.
public ActionResult UploadImages(FormCollection collection) { ApiResponse response = new ApiResponse(); HttpFileCollectionBase files = Request.Files; byte[] data; string Name = collection["txtName"]; string Address = collection["txtAddress"]; string Description = collection["txtDescription"]; if (string.IsNullOrEmpty(Name)) { response.msg = "Please enter Title.."; return View(response); } if (string.IsNullOrEmpty(Address)) { response.msg = "Please enter Address.."; return View(response); } MultipartFormDataContent multiForm = new MultipartFormDataContent(); multiForm.Add(new StringContent(Name), "Name"); multiForm.Add(new StringContent(Address), "Address"); multiForm.Add(new StringContent(Description), "Description"); multiForm.Add(new StringContent("343"), "UserId"); if (files.Count > 0) { //adding list of images in the MultipartFormDataContent with same key for (int i = 0; i < files.Count; i++) { HttpPostedFileBase file = files[i]; if (file.ContentLength > 0) { ByteArrayContent bytes; using (var br = new BinaryReader(file.InputStream)) { data = br.ReadBytes((int)file.InputStream.Length); } bytes = new ByteArrayContent(data); multiForm.Add(bytes, "Images", "filename.jpg"); } } HttpClient client = new HttpClient(); client.BaseAddress = new Uri("http://localhost:44246/"); client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json")); HttpResponseMessage httpResponseMessage = client.PostAsync("/api/Profileupdate/Uploadimages", multiForm).Result; if (httpResponseMessage.IsSuccessStatusCode) { //string ss = httpResponseMessage.StatusCode.ToString(); response = httpResponseMessage.Content.ReadAsAsync<ApiResponse>().Result; } else { var content = httpResponseMessage.Content.ReadAsStringAsync().Result; response.msg = content; //string str = await httpResponseMessage.Content.ReadAsStringAsync(); ; } return View(); } else { return View(); } }
I hope it clarifies your concern if you have any doubt please comment.
Thank you..
This UploadImages action method handles the uploading of images along with additional form data to a web API.
- The method accepts a FormCollection object, which contains the form data submitted by the client.
- It initializes an ApiResponse object to store the response data.
- It retrieves the files uploaded by the client using Request.Files.
- It retrieves the form data such as Name, Address, and Description from the collection.
- It validates the form data, ensuring that Name and Address are not empty. If either of them is empty, it sets an appropriate message in the response object and returns the view with the response.
- It constructs a MultipartFormDataContent object to hold both the form data and the images.
- It adds the Name, Address, Description, and UserId (set to "343") to the multiForm object.
- If files are uploaded, it iterates through each file, reads its content as a byte array, and adds it to the multiForm object along with the key "Images".
- It initializes an HttpClient object and sets its base address to the URL of the web API.
- It sends a POST request to the web API endpoint ("/api/Profileupdate/Uploadimages") with the multiForm content.
- If the response from the web API indicates success (HTTP status code 200), it reads the response content and assigns it to the response object.
- If the response indicates failure, it reads the error message from the response content and sets it in the response object.
- It returns the view without any model if there are no files uploaded, or with the response message otherwise.
This function provide code for uploading of images and form data to a web API endpoint using the HTTP client.
Because this file that we have here must match this file that we have here. It is the name of the parameter now. Com. And here we want to put the file name. Now I will say wait step client do post sync and I want to pass URL CMA request content and that’s actually
With this, we’re good to go. Let me first make sure that in www. Root, there is nothing right. So now let me press control five to run our application, both the console application and also the API. As you can see the end, means that we finish.
So let’s go back here and let’s see what we have. Let’s go to the Solution Explorer. In the www. Directory, we have a file folder. And in here we have these text file that has a Git as its name.
That is because I don’t like to leave the same name on the file because, in the future, we could have collisions in the name of the file. But if I double click here, you are going to see that we have this is an example which is the same content that our example TXT file has. Therefore, as you can see, we were able to send a file from a client to our API by using the HTTP client class.
Read Similar Articles
- How can we refresh a table using AJAX in ASP.NET MVC?
- React Js-Insert Update Display Delete CRUD Operations
- [Solved]:Unable to create an object of type 'DbContext'. For the different patterns supported at design time
- [Simple Way]-How To Display Array Data Into Tables In Reactjs
- How To Inject Dependency To Static Class In C#
- [Solved]-Cookie Loses Value When Page Is Changed In MVC
- Scalar User-defined functions In Sql Server-Step by Step